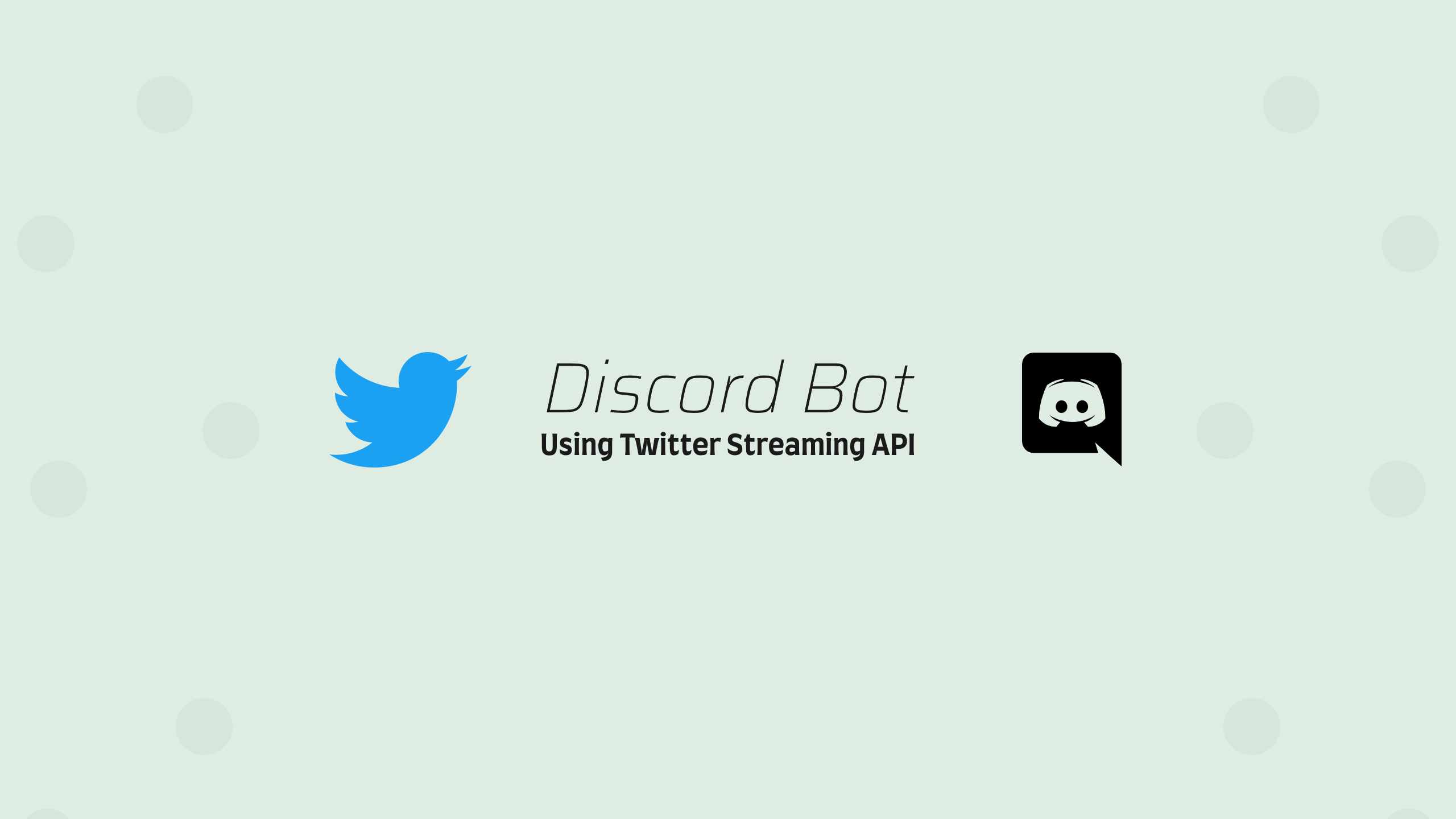
UPDATES FOR V2 TWITTER API!!!!
Discord Is Awesome, So Is Twitter
I have a discord server and I have a pretty decent sized Twitter following. I wanted to add a bot that followed my tweets and sent them as messages in my #twitter channel on my server. I refuse to pay for software and I have a hyper distrust of other’s software so I wrote my own. In this node.js script I have one file main.js that uses 3 dependencies: twit (for Twitter streaming API), Discord.js (for well….Discord) and dotenv (to load environment variables). The script is ~30 lines long and is available on my Github.
Main.js
require('dotenv').config()
const Twit = require('twit')
const Discord = require('discord.js');
const client = new Discord.Client();
var T = new Twit({
consumer_key: process.env.TWITTER_CONSUMER_KEY,
consumer_secret: process.env.TWITTER_CONSUMER_SECRET,
access_token: process.env.TWITTER_ACCESS_TOKEN,
access_token_secret: process.env.TWITTER_ACCESS_TOKEN_SECRET,
timeout_ms: 60*1000, // optional HTTP request timeout to apply to all requests.
strictSSL: true, // optional - requires SSL certificates to be valid.
})
client.login(process.env.DISCORD_TOKEN);
client.once('ready', () => {
var stream = T.stream('statuses/filter', { follow: [process.env.TWITTER_USER_ID] })
stream.on('tweet', function (tweet) {
//...
var url = "https://twitter.com/" + tweet.user.screen_name + "/status/" + tweet.id_str;
try {
let channel = client.channels.fetch(process.env.DISCORD_CHANNEL_ID).then(channel => {
channel.send(url)
}).catch(err => {
console.log(err)
})
} catch (error) {
console.error(error);
}
})
})
.env file to load variables.
TWITTER_CONSUMER_KEY=
TWITTER_CONSUMER_SECRET=
TWITTER_ACCESS_TOKEN=
TWITTER_ACCESS_TOKEN_SECRET=
TWITTER_USER_ID=
DISCORD_TOKEN=
DISCORD_CHANNEL_ID=
Now all you have to do is run it using the following command
node main.js
Don’t forget to get your application keys from the Discord developer portal and the Twitter developer portal. I made a Youtube video showing the process in detail. Want to start making money and living a #CodeLife? Join the group today!