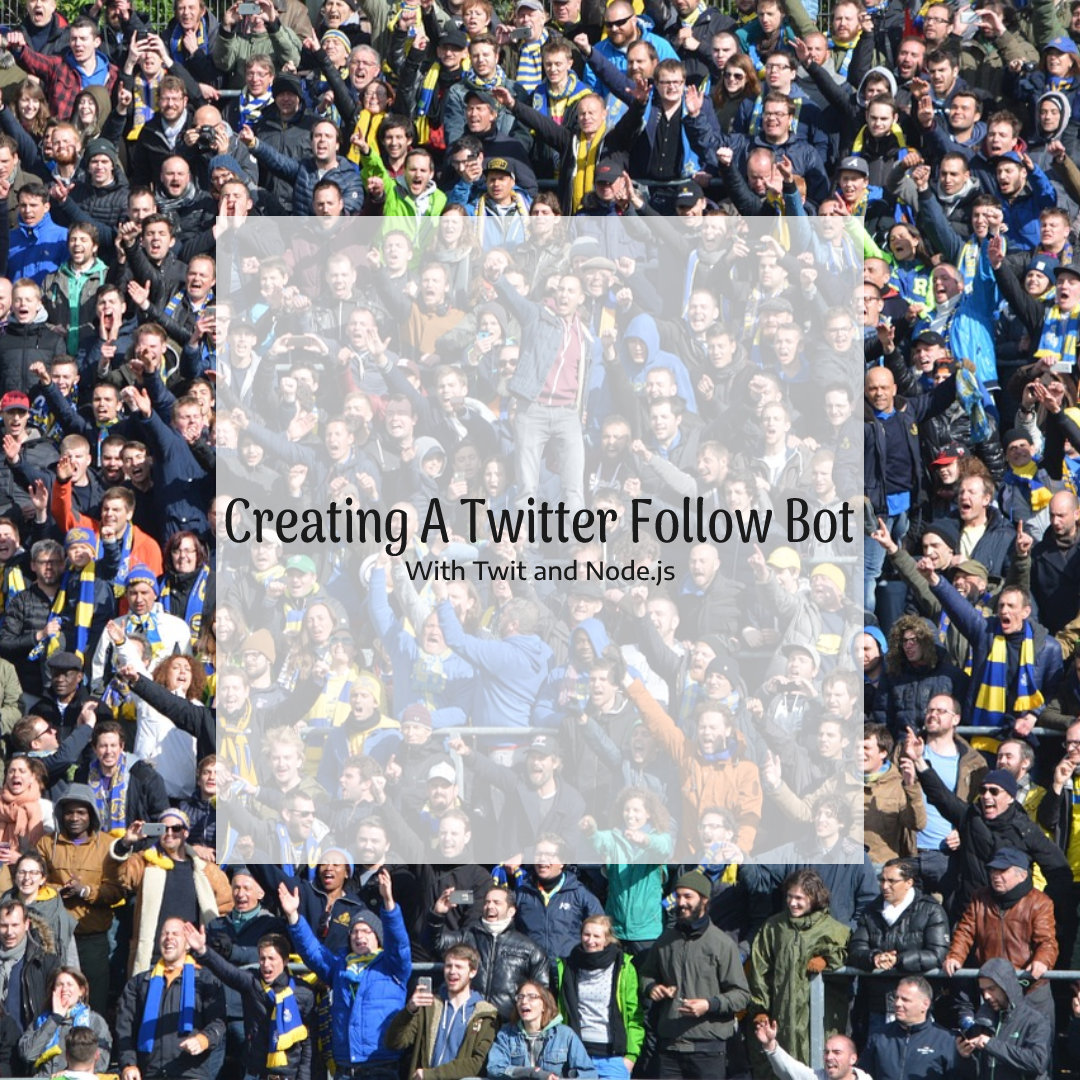
Automate Your Following With A Twitter Follow Bot
Anyone who follows me on Twitter (if you don’t @mastashake08) knows that I’m pretty active. Currently I’m on my way to 10K followers but sometimes my TL looks kinda dry. One of the best things about Twitter is that I learn alot of new information from the people I follow. Yet I don’t have the time to actively look for new people. Time for a #CodeLife trick.
Filtering Statuses With Twit
If you read my article on Creating a Discord Twitter Bot then alot of this code will look familiar to you. I’m going to stream a list of filtered statuses that use the hashtags #BlackTechTwitter and #CodeLife and whoever sends those tweets I will automatically follow them. So let’s begin by creating a new directory and adding our dependecies
mkdir follow-bot
cd follow-bot && npm install dotenv twit
touch search-follow.js
This will create a new directory, cd into it and install the dotenv and twit dependencies. Dotenv allows us to use a .env file to hold our secret values for our Twitter creditenials safely and twit is the Twitter javascript library. Lastly we created an empty js file where we will hold our code. Open it up and input the following.
require('dotenv').config()
const Twit = require('twit')
var T = new Twit({
consumer_key: process.env.TWITTER_CONSUMER_KEY,
consumer_secret: process.env.TWITTER_CONSUMER_SECRET,
access_token: process.env.TWITTER_ACCESS_TOKEN,
access_token_secret: process.env.TWITTER_ACCESS_TOKEN_SECRET,
timeout_ms: 60*1000, // optional HTTP request timeout to apply to all requests.
strictSSL: true, // optional - requires SSL certificates to be valid.
})
var stream = T.stream('statuses/filter', { track: ['#blacktechtwitter', '#codelife'], language: 'en' })
stream.on('tweet', function (tweet) {
//...
var user = tweet.user;
try {
T.post('friendships/create', {screen_name: user.screen_name})
console.log('Followed ' + user.screen_name)
} catch (error) {
console.log(error)
}
})
The first lines we are requiring our dependencies and after we initialize the Twit object with out Twitter API creds stored in .env
Next we set a stream variable that will hold our filtered statuses tracking the list of hashtags.
Since the stream is event-driven we listen for the ‘tweet’ event which holds our Tweet object. We grab the screen_name of the user who tweeted and make a request to the ‘friendships/create’ endpoint which is what creates the following!
See how simple that was! If you enjoyed this article consider becoming a patron to get exclusive content! Get the source code here.