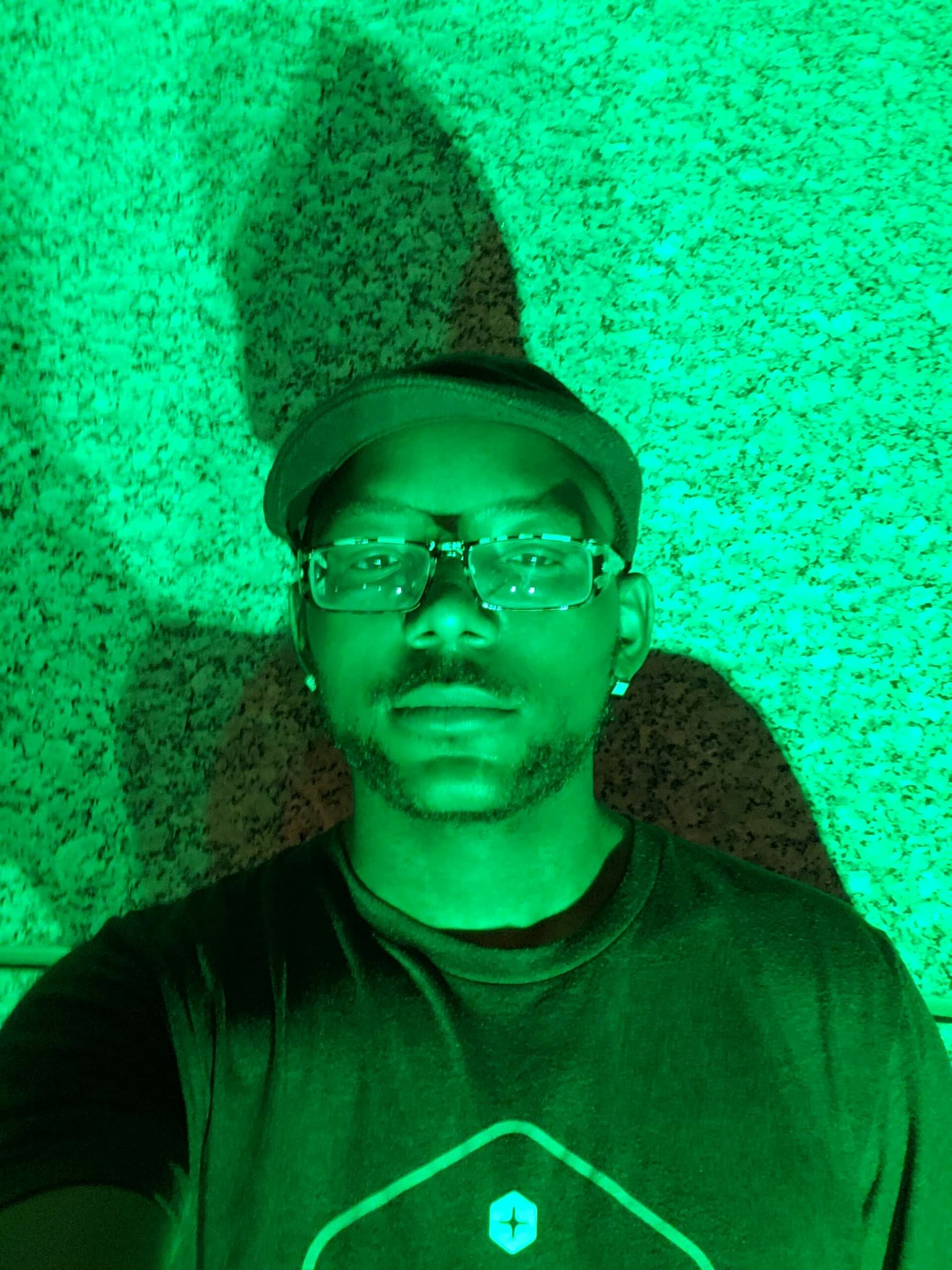
[callaction url=”https://www.youtube.com/user/JPlaya01″ background_color=”#333333″ text_color=”#ffffff” button_text=”Go Now” button_background_color=”#e64429″]Subscribe To My Youtube Page[/callaction]
Ionic
I figured Ionic would be a great choice for creating the mobile chat app. This chat app is dirt simple:
- Open up a websocket connection to the Lumen service and listen for messages
- Post messages to Lumen Service
Follow along, I won’t be adding any target platforms, just testing in browser. However if you want to port this that another platform just follow this guide. Let’s start! If you don’t already have the software installed type in this command npm -g install cordova ionic
you may have to run this command as sudo
. Afterwards cd into an empty directory and run this command
ionic start messenger-mobile blank
. It will go through the process of creating a new Ionic application, when complete cd into your new app.
Dependencies
Now we need to install our dependencies first off we need to install the socket.io-client to communicate with our real-time chat server. This is available via npm, type in this command
npm install socket.io-client
and copy the folder
node_modules/socket.io-client
to the lib folder (that’s where everything else is ionic related). Next type in this command
ionic plugin add cordova-plugin-whitelist
, this in needed because we have to access remote resources, and need to whitelist them.
Whitelisting
Open up the config.xml file at the root of your application and add this line
<meta http-equiv="Content-Security-Policy" content="default-src *; style-src 'self' 'unsafe-inline'; script-src 'self' 'unsafe-inline' 'unsafe-eval'">
This allows us to go to any remote resource. Now we won’t get any errors trying to access our websocket. Now lets create our WebSocket factory.
WebSocket Factory
Create a seperate file in your www/js folder called services.js. Inside put the following code:
angular.module('services', []) .factory('WebSocket', function($http) { // Open a WebSocket connection var dataStream = io('http://messenger.app:6001');
console.log(dataStream);
var messages = [];
dataStream.on('message',function(data) {messages.push(data.message); console.log(messages);
});var methods = { messages: messages, send: function(message) { $http.post('http://messenger.app/message',{ message:message}).success(function(data){ ///messages.push(data); //console.log(messages); }); } };
return methods; });
Change http://messenger.app to the IP address of your microservice. Right now this code would break because we haven’t included the io dependecy but we will get to that.
App.js
Open www/js/app.js this is our main JS file, we are going to include our services file in here and access the WebSocket factory. Copy this code:
// Ionic Starter App
// angular.module is a global place for creating, registering and retrieving Angular modules // 'starter' is the name of this angular module example (also set in a <body> attribute in index.html) // the 2nd parameter is an array of 'requires'angular.module('real-time-messaging', ['ionic','services'])
.run(function($ionicPlatform) {$ionicPlatform.ready(function() { // Hide the accessory bar by default (remove this to show the accessory bar above the keyboard // for form inputs) if(window.cordova && window.cordova.plugins.Keyboard) { cordova.plugins.Keyboard.hideKeyboardAccessoryBar(true); } if(window.StatusBar) { StatusBar.styleDefault(); } }); })
.controller('HomeController',function($scope,WebSocket){ $scope.socket = WebSocket; console.log($scope.socket); $scope.messageModel = {}; $scope.sendMessage = function(){ console.log('SEND MESSAGE PUSHED!'); console.log('Message Sent ' + $scope.messageModel.message); $scope.socket.send($scope.messageModel.message); console.log($scope.socket.messages); }; });
The code is pretty self explanatory, we have a single function in our HomeController that sends a message to the microservice. We have all the real time logic encapsulated in the WebSocket factory, so we are complete here!
Index.html
Lastly we need to modify the index.html. For simplicity sake this page only has a list to hold messages and a form to post a message. Copy the following code:
<!DOCTYPE html> <html>
<head> <meta charset="utf-8"> <meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no, width=device-width"> <title></title> <script src="lib/socket.io-client/socket.io.js"></script> <link href="lib/ionic/css/ionic.css" rel="stylesheet"> <link href="css/style.css" rel="stylesheet">
<!-- IF using Sass (run gulp sass first), then uncomment below and remove the CSS includes above <link href="css/ionic.app.css" rel="stylesheet"> -->
<!-- ionic/angularjs js --> <script src="lib/ionic/js/ionic.bundle.js"></script>
<!-- cordova script (this will be a 404 during development) --> <script src="cordova.js"></script>
<!-- your app's js --> <script src="js/app.js"></script>
<!-- your app's js --> <script src="js/services.js"></script>
</head> <body ng-app="real-time-messaging" ng-controller="HomeController">
<ion-pane> <ion-header-bar class="bar-stable"> <h1 class="title">Real Time Messaging</h1> </ion-header-bar> <ion-content> <textarea ng-model="messageModel.message" placeholder="Enter Message"></textarea> <br> <button ng-click="sendMessage()" class="icon icon-left ion-paper-airplane button button-positive"> Send Message </button>
<ion-list> <ion-item ng-repeat="message in socket.messages track by $index"> Messages: {{message}} </ion-item> </ion-list> </ion-content> </ion-pane> </body> </html>
The app is complete! You can test it in the browser by typing ionic serve
in the terminal, or you can add a platform and test on an emulator or physical device. If you have any questions, or want to see some future enhancements, please comment below!