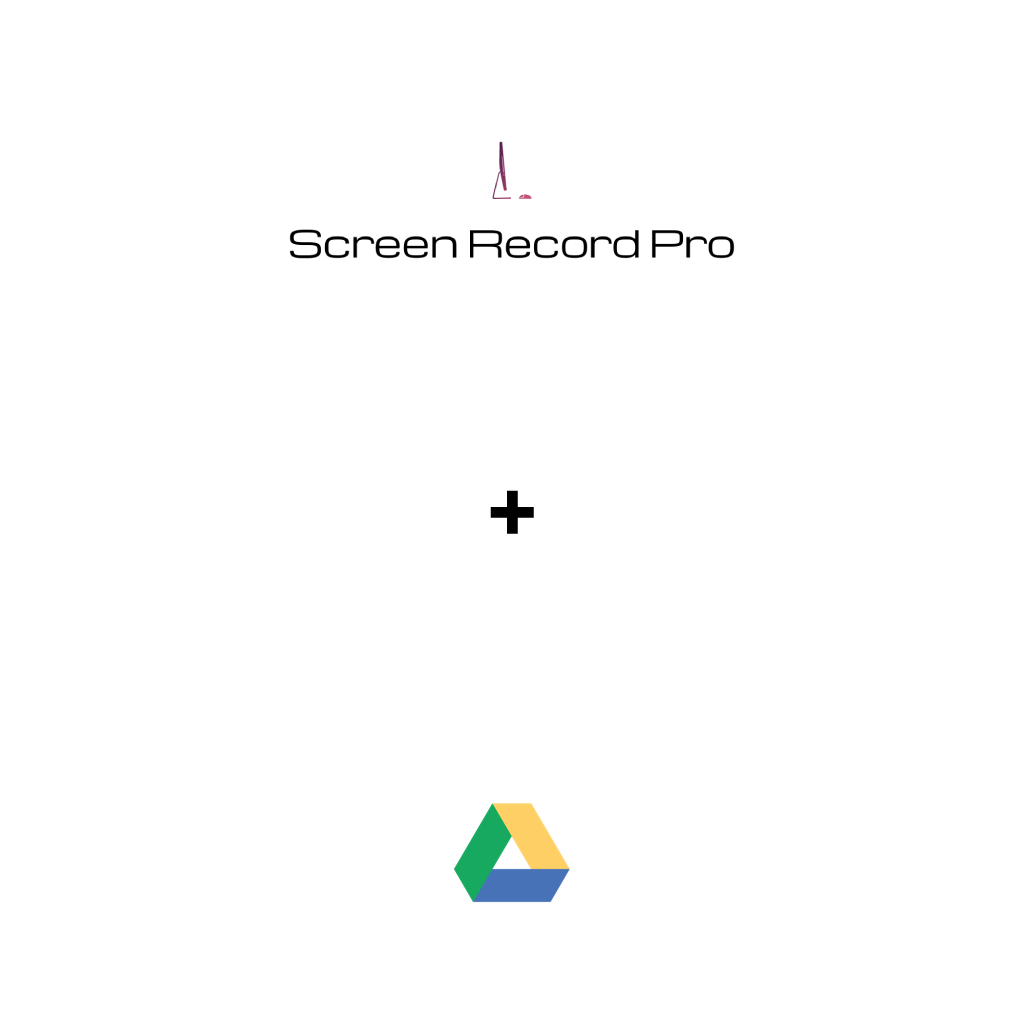
You All Requested Google Drive Functionality!
In my last YouTube video, I was asked to implement Google Drive upload functionality for saving screen recordings. I thought this was a marvelous idea and immediately got to work! We already added OAuth login via Google and Laravel in the last tutorial to interact with the Youtube Data v3 API, so with a few simple backend tweaks, we can add Google Drive as well!
Steps To Accomplish
The functionality I want to add to this is going to be just uploading to Google Drive, with no editing or listing. Keep things simple! This is going to require the following steps
- Adding Google Drive scopes to Laravel Socialite
- Create a function to upload file to Google API endpoint
Pretty easy if I do say so myself. Let’s get started with the backend.
Adding Google Drive Scopes To Laravel Socialite
We already added scopes for YouTube in the last tutorial so thankfully not a whole lot of work is needed to add Google Drive scopes. Open up your routes/api.php file and update the scopes array to include the new scopes needed to interact with Google Drive
Route::get('/login/youtube', function (Request $request) {
return Socialite::driver('youtube')->scopes(['https://www.googleapis.com/auth/youtube', 'https://www.googleapis.com/auth/youtube.upload', 'https://www.googleapis.com/auth/youtube.readonly', 'https://www.googleapis.com/auth/drive', 'https://www.googleapis.com/auth/drive.metadata', 'https://www.googleapis.com/auth/drive.metadata.readonly'])->stateless()->redirect();
});
Make sure you enable the API in the Google cloud console! Now we head over to the frontend Vue application and let’s add our markup and functions.
Open the Home.vue and we are going to add a button in our list of actions for uploading to Google Drive
<t-button v-on:click="uploadToDrive" v-if="uploadReady" class="ml-10">Upload To Drive 🗄️</t-button>
In the methods add a function called uploadToDrive() inside put the following
async uploadToDrive () {
let metadata = {
'name': 'Screen Recorder Pro - ' + new Date(), // Filename at Google Drive
'mimeType': 'application/zip', // mimeType at Google Drive
}
let form = new FormData();
form.append('metadata', new Blob([JSON.stringify(metadata)], {type: 'application/json'}));
form.append('file', this.file);
await fetch('https://www.googleapis.com/upload/drive/v3/files?uploadType=multipart', {
method: 'POST', // *GET, POST, PUT, DELETE, etc.
mode: 'cors', // no-cors, *cors, same-origin
cache: 'no-cache',
headers: {
'Content-Length': this.file.length,
Authorization: `Bearer ${this.yt_token}`
},
body: form
})
alert('Video uploaded to Google Drive!')
}
Inside this function we create an HTTP POST request to the Google Drive endpoint for uploading files. We pass a FormData object that contains some metadata about the object and the actual file itself. After the file is uploaded the user is alerted that their video is stored!
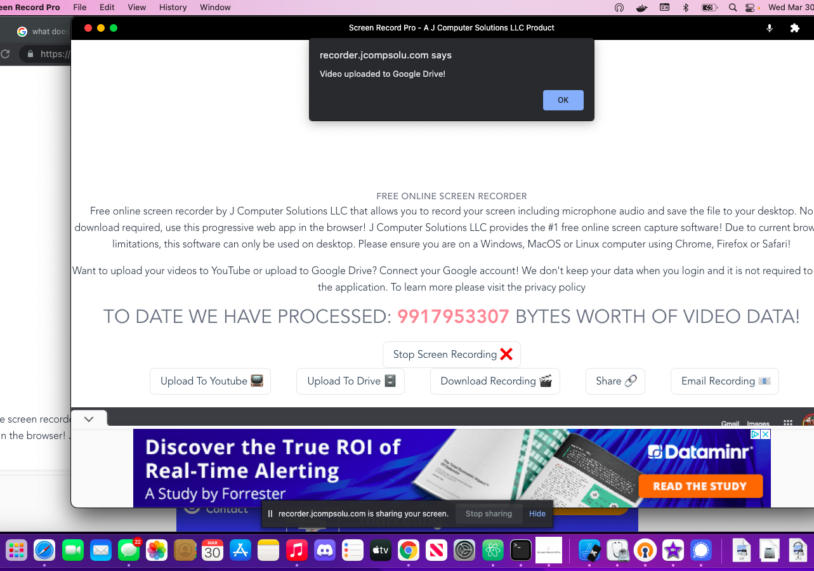
What’s Next?
Next, we will add cloud storage you will be able to share with Amazon S3 and WebShare API! Finally we will add monetization and this project will be wrapped up! If you enjoyed this please give the app a try at https://recorder.jcompsolu.com